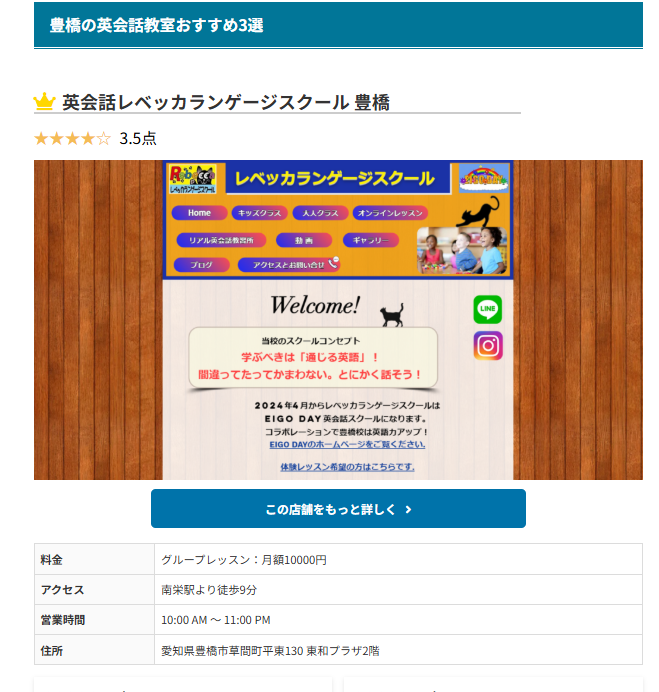
外部リンクです
目次
追加したカスタムフィールド
タイプ | ラベル | 名前 |
テキスト | レビューコメント | review_comment |
php
function show_area_ranking_block($post_id) {
$categories = get_the_category($post_id);
if (empty($categories)) return '';
$category = $categories[0];
$args = array(
'post_type' => 'post',
'posts_per_page' => 3,
'category__in' => array($category->term_id),
'meta_key' => 'review_score',
'orderby' => 'meta_value_num',
'order' => 'DESC',
);
$query = new WP_Query($args);
if (!$query->have_posts()) return '';
ob_start();
echo '<div class="ranking-section">';
echo '<h2>' . esc_html($category->name) . 'おすすめ3選</h2>';
$rank = 1;
while ($query->have_posts()) {
$query->the_post();
$post_id = get_the_ID();
$salon_name = esc_html(SCF::get('salon_name', $post_id));
$score = floatval(SCF::get('review_score', $post_id));
$main_banner_id = SCF::get('main_banner', $post_id);
$main_banner = wp_get_attachment_image_url($main_banner_id, 'large');
$price = esc_html(SCF::get('budget', $post_id));
$access = esc_html(SCF::get('access', $post_id));
$hours = esc_html(SCF::get('business_hours', $post_id));
$address = esc_html(SCF::get('address', $post_id));
$reviews = SCF::get('口コミ', $post_id);
echo '<div class="ranking-item">'; // ← スペース用の囲み
// 順位に応じた色設定
$crown_color = '#FFD700'; // gold
if ($rank === 2) $crown_color = '#C0C0C0'; // silver
if ($rank === 3) $crown_color = '#CD7F32'; // bronze
echo '<div class="ranking-header" style="display: flex; align-items: center; gap: 8px; margin-bottom: 12px; padding-bottom: 8px;">';
echo '<i class="fas fa-crown" style="color:' . esc_attr($crown_color) . '; font-size: 1.5rem;"></i>';
echo '<span class="salon-name" style="font-size: 1.2rem; font-weight: bold;">' . $salon_name . '</span>';
echo '</div>';
echo '<div class="review-stars">★★★★☆ <span>' . number_format($score, 1) . '点</span></div>';
echo '<div class="ranking-image"><img src="' . esc_url($main_banner) . '" alt="" class="main-banner-image" style="width:100%; height:auto; display:block;"></div>';
echo '<a href="' . get_permalink($post_id) . '" class="details-button">この店舗をもっと詳しく <i class="fas fa-angle-right"></i></a>';
echo '<table class="info-table">';
echo '<tr><th>料金</th><td>' . $price . '</td></tr>';
echo '<tr><th>アクセス</th><td>' . $access . '</td></tr>';
echo '<tr><th>営業時間</th><td>' . $hours . '</td></tr>';
echo '<tr><th>住所</th><td>' . $address . '</td></tr>';
echo '</table>';
echo '<div class="reviews-carousel">';
if (is_array($reviews)) {
$i = 0;
foreach ($reviews as $review) {
if ($i >= 2) break;
$review_score = floatval($review['review_number']);
$review_text = esc_html($review['review_text']);
echo '<div class="review-item">';
echo '<div class="review-rating">★★★★☆ <span>' . number_format($review_score, 1) . '点</span></div>';
echo '<p class="review-text">' . $review_text . '</p>';
echo '</div>';
$i++;
}
}
echo '</div>'; // .reviews-carousel
echo '</div>'; // .ranking-item
$rank++;
}
echo '</div>';
wp_reset_postdata();
return ob_get_clean();
}
add_shortcode('area_ranking_block', function () {
return show_area_ranking_block(get_the_ID());
});
CSS
/* 店舗ページ記事下ランキング表示*/
.ranking-section {
margin: 20px 0;
}
/* 王冠と見出し */
.ranking-header {
display: flex;
align-items: center;
gap: 8px;
/* 調整可能な余白エリア */
margin-top: 0px; /* 👈 線の上に余白を作りたいとき調整 */
margin-bottom: 12px; /* 👈 線の下に余白を作りたいとき調整 */
padding-bottom: 10px; /* 👈 線の表示位置の下押し調整 */
overflow: visible; /* ← これを指定してみてください */
position: relative;
}
.ranking-header::after {
content: "";
display: block;
position: absolute;
bottom: 0;
left: 0;
width: 80% !important;
height: 2px;
background-color: #ccc;
}
.ranking-header i.fa-crown {
font-size: 1.6rem;
display: inline-block;
position: relative;
top: 10px;
}
.salon-name {
font-size: 1.3rem;
font-weight: bold;
line-height: 1.2;
}
/* 評価表示(見出し下) */
.review-stars {
font-size: 1.3rem;
color: #F5BC55;
margin: 4px 0 12px;
}
.review-stars span {
color: #000; /* 👈 点数は黒に */
margin-left: 6px;
}
/* 口コミエリア */
.reviews-carousel {
display: flex;
flex-wrap: wrap;
gap: 16px;
margin-top: 16px;
}
.review-item {
background-color: #FFFFFF;
box-shadow: 0 2px 6px rgba(0, 0, 0, 0.1);
padding: 12px;
flex: 1 1 calc(50% - 8px);
}
.review-rating {
font-weight: bold;
margin-bottom: 4px;
color: #F5BC55; /* ★は黄色 */
}
.review-rating span {
color: #000; /* 点数は黒 */
}
.review-text {
font-size: 0.95em;
}
/* スマホ対応(1列に) */
@media (max-width: 768px) {
.review-item {
flex: 1 1 100%;
}
}
.details-button {
display: block;
width: 80%;
max-width: 500px; /* 任意:大画面でも広がりすぎないように */
margin: 20px auto; /* ← 上下マージン20px、左右autoで中央寄せ */
box-sizing: border-box;
background-color: #0073aa;
color: #fff;
padding: 12px 20px;
font-size: 1rem;
text-align: center;
text-decoration: none;
border-radius: 6px;
font-weight: bold;
margin-bottom: 20px;
transition: background-color 0.3s ease;
border: none;
/* ⬇⬇⬇ ここが調整ポイント ⬇⬇⬇ */
margin-top: 12px; /* ボタンの上の余白 */
margin-bottom: 20px; /* ボタンの下の余白 */
}
.details-button:hover {
background-color: #005f8d;
}
.details-button i {
margin-left: 8px;
}
.main-banner-image {
width: 100%;
height: auto;
display: block;
margin-bottom: 20px; /* 下に余白をつけたい場合 */
}
.ranking-item {
margin-bottom: 32px; /* 👈 ランキング項目ごとのスペース */
}
ショートコード
[area_ranking_block]
コメント