目次
JSの配置
click-tracker.js の中身
jQuery(document).ready(function () {
jQuery('body').on('click', 'a.swell-block-button__link', function (e) {
var parent = jQuery(this).closest('.track-click');
if (parent.length === 0) return; // track-click クラスが親にないなら無視
e.preventDefault(); // 通常のクリック遷移を止める
e.stopImmediatePropagation(); // SWELLや他JSのイベントも止める
console.log('クリックされた:処理開始');
var url = jQuery(this).attr('href');
var id = clickTrackerAjax.content_type === 'term' ? clickTrackerAjax.term_id : clickTrackerAjax.post_id;
var type = clickTrackerAjax.content_type;
jQuery.post(clickTrackerAjax.ajax_url, {
action: 'custom_click_track',
id: id,
type: type
}).done(function(res) {
console.log('クリックカウント送信成功', res);
console.log('クリックID', id, 'タイプ', type);
}).fail(function(err) {
console.error('クリックカウント送信失敗', err);
}).always(function () {
window.location.href = url;
});
return false;
});
});
reset-stats.js の中身
jQuery(document).on('click', '.reset-stats-button', function (e) {
e.preventDefault();
if (!confirm('このページのカウントをリセットしますか?')) return;
const id = jQuery(this).data('id');
const type = jQuery(this).data('type');
jQuery.post(ajaxurl, {
action: 'reset_stats',
id: id,
type: type
}, function (response) {
alert('リセットしました。再読み込みしてください。');
});
});
Code SnipetにてPHP作成(【全体】ページごとの閲覧数を保存)
// ① 閲覧数をSCFに記録(投稿・固定ページ・カテゴリーページに対応)
function custom_count_page_view() {
if (is_admin()) return;
if (current_user_can('manage_options')) return; // 管理者を除外
if (is_single() || is_page()) {
$post_id = get_the_ID();
$meta_key = 'page_views';
$views = get_post_meta($post_id, $meta_key, true);
$views = is_numeric($views) ? (int)$views + 1 : 1;
update_post_meta($post_id, $meta_key, $views);
} elseif (is_category()) {
$term_id = get_queried_object_id();
$meta_key = 'page_views';
$views = get_term_meta($term_id, $meta_key, true);
$views = is_numeric($views) ? (int)$views + 1 : 1;
update_term_meta($term_id, $meta_key, $views);
}
}
add_action('wp', 'custom_count_page_view');
// ② 外部リンククリックを記録(Ajax処理)
function custom_enqueue_click_tracking_script() {
wp_enqueue_script(
'click-tracker',
get_stylesheet_directory_uri() . '/js/click-tracker.js',
['jquery'],
null,
true
);
$post_id = is_singular() ? get_the_ID() : 0;
$term_id = is_category() ? get_queried_object_id() : 0;
wp_localize_script('click-tracker', 'clickTrackerAjax', [
'ajax_url' => admin_url('admin-ajax.php'),
'post_id' => $post_id,
'term_id' => $term_id,
'content_type' => is_category() ? 'term' : 'post'
]);
}
add_action('wp_enqueue_scripts', 'custom_enqueue_click_tracking_script');
function custom_ajax_track_click() {
if (current_user_can('manage_options')) wp_die(); // 管理者を除外
$type = sanitize_text_field($_POST['type']);
$id = intval($_POST['id']);
if ($type === 'post') {
$meta_key = 'click_count';
$existing = get_post_meta($id, $meta_key, true);
$new_val = is_numeric($existing) ? (int)$existing + 1 : 1;
update_post_meta($id, $meta_key, $new_val);
} elseif ($type === 'term') {
$term_id = 'term_' . $id;
$meta_key = 'click_count';
$existing = get_term_meta($term_id, $meta_key, true);
$new_val = is_numeric($existing) ? (int)$existing + 1 : 1;
update_term_meta($term_id, $meta_key, $new_val);
}
wp_die();
}
add_action('wp_ajax_custom_click_track', 'custom_ajax_track_click');
add_action('wp_ajax_nopriv_custom_click_track', 'custom_ajax_track_click');
// ③ WordPressの管理画面に「アクセス統計」ページを追加
add_action('admin_menu', function() {
add_menu_page(
'アクセス統計',
'アクセス統計',
'manage_options',
'custom-access-stats',
'render_custom_access_stats_page',
'dashicons-chart-bar',
30
);
});
function render_custom_access_stats_page() {
echo '<div class="wrap"><h1>アクセス統計</h1>';
// ヒント表示
echo '<div class="notice notice-info" style="margin-bottom:20px;padding:15px;border-left:4px solid #00a0d2;background:#f1f9ff;">
<strong>💡クリックカウントを有効にするには:</strong><br>
外部リンクの<a>タグの親要素に <code>track-click</code> クラスを付けてください。<br>
</div>';
// 投稿・固定ページ
$post_types = ['post', 'page'];
$args = [
'post_type' => $post_types,
'posts_per_page' => -1,
'post_status' => 'publish',
];
$posts = get_posts($args);
// 閲覧数で降順ソート
usort($posts, function($a, $b) {
$views_a = (int) get_post_meta($a->ID, 'page_views', true);
$views_b = (int) get_post_meta($b->ID, 'page_views', true);
return $views_b - $views_a;
});
echo '<h2>投稿・固定ページ</h2>';
echo '<table class="widefat"><thead><tr><th>タイトル</th><th>閲覧数</th><th>クリック数</th><th>クリック率</th><th>リセット</th><th>リセット日</th></tr></thead><tbody>';
foreach ($posts as $post) {
$views = (int) get_post_meta($post->ID, 'page_views', true);
$clicks = (int) get_post_meta($post->ID, 'click_count', true);
$reset_date = get_post_meta($post->ID, 'reset_date', true);
$rate = ($views > 0) ? round(($clicks / $views) * 100, 1) . '%' : '–';
echo '<tr>';
echo '<td><a href="' . get_permalink($post->ID) . '" target="_blank">' . esc_html(get_the_title($post->ID)) . '</a></td>';
echo '<td>' . $views . '</td>';
echo '<td>' . $clicks . '</td>';
echo '<td>' . $rate . '</td>';
echo '<td><button class="reset-stats-button" data-id="' . esc_attr($post->ID) . '" data-type="post">リセット</button></td>';
echo '<td>' . esc_html($reset_date ?: '—') . '</td>';
echo '</tr>';
}
echo '</tbody></table>';
// カテゴリーページ
$terms = get_terms([
'taxonomy' => 'category',
'hide_empty' => false,
]);
// 閲覧数で降順ソート
usort($terms, function($a, $b) {
$views_a = (int) get_term_meta($a->term_id, 'page_views', true);
$views_b = (int) get_term_meta($b->term_id, 'page_views', true);
return $views_b - $views_a;
});
echo '<h2>カテゴリーページ</h2>';
echo '<table class="widefat"><thead><tr><th>カテゴリ名</th><th>閲覧数</th><th>クリック数</th><th>クリック率</th><th>リセット</th><th>リセット日</th></tr></thead><tbody>';
foreach ($terms as $term) {
$views = (int) get_term_meta($term->term_id, 'page_views', true);
$clicks = (int) get_term_meta($term->term_id, 'click_count', true);
$reset_date = get_term_meta($term->term_id, 'reset_date', true);
$rate = ($views > 0) ? round(($clicks / $views) * 100, 1) . '%' : '–';
echo '<tr>';
echo '<td><a href="' . get_category_link($term->term_id) . '" target="_blank">' . esc_html($term->name) . '</a></td>';
echo '<td>' . $views . '</td>';
echo '<td>' . $clicks . '</td>';
echo '<td>' . $rate . '</td>';
echo '<td><button class="reset-stats-button" data-id="' . esc_attr($term->term_id) . '" data-type="term">リセット</button></td>';
echo '<td>' . esc_html($reset_date ?: '—') . '</td>';
echo '</tr>';
}
echo '</tbody></table>';
echo '</div>';
}
function custom_ajax_reset_stats() {
if (!current_user_can('manage_options')) wp_die('権限がありません');
$id = intval($_POST['id']);
$type = sanitize_text_field($_POST['type']);
$today = date('Y-m-d');
if ($type === 'post') {
update_post_meta($id, 'page_views', 0);
update_post_meta($id, 'click_count', 0);
update_post_meta($id, 'reset_date', $today);
} elseif ($type === 'term') {
update_term_meta($id, 'page_views', 0);
update_term_meta($id, 'click_count', 0);
update_term_meta($id, 'reset_date', $today);
}
wp_die('OK');
}
add_action('wp_ajax_reset_stats', 'custom_ajax_reset_stats');
add_action('admin_enqueue_scripts', function () {
wp_enqueue_script(
'reset-stats',
get_stylesheet_directory_uri() . '/js/reset-stats.js',
['jquery'],
null,
true
);
});
リンク計測するボタンを以下のように設置
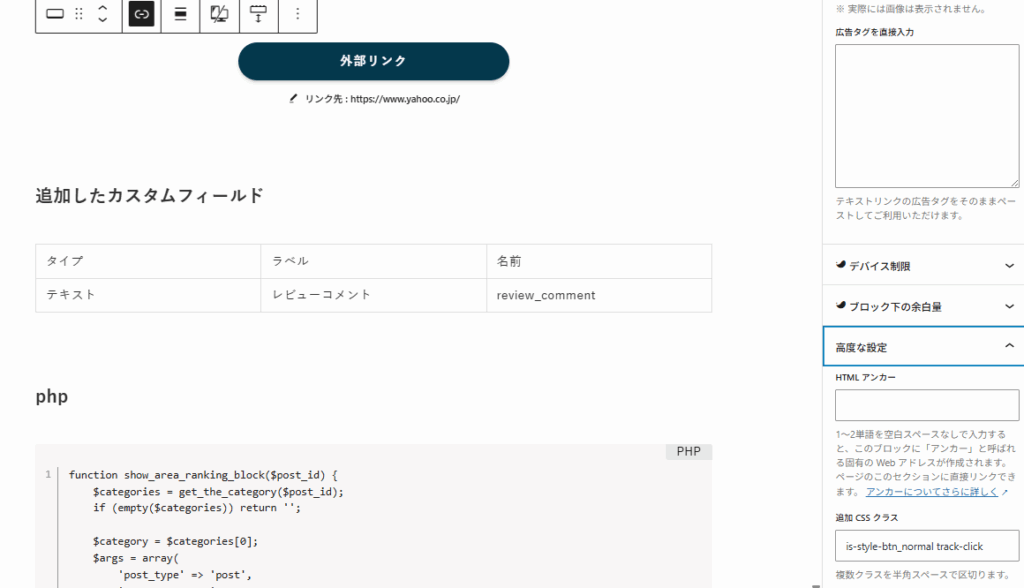
コメント